本篇旨在记载STL一些常见函数及用法以备记忆
栈容器 stack
用法:
- empty() 堆栈为空则返回真
- pop() 移除栈顶元素
- push() 在栈顶增加元素
- size() 返回栈中元素数目
- top() 返回栈顶元素
初始化: stack<int> s
示例:简单计算器
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96
| #include <iostream> #include <string> #include <stack> #include <algorithm> using namespace std; stack<char> op; stack<float> num;
float cal(float num1, float num2, char op) { if (op == '+') return num1 + num2; else if (op == '-') return num2 - num1; else if (op == '*') return num2 * num1; else if (op == '/') return num2 / num1; } int main() { string s; while (cin >> s && s != "0") { for (float i = 0; i < s.length(); i++) { if (s[i] == '+' || s[i] == '-') { while (!op.empty()) { char tmpop = op.top(); op.pop(); float num1 = num.top(); num.pop(); float num2 = num.top(); num.pop(); float calnum = cal(num1, num2, tmpop); num.push(calnum); }
op.push(s[i]); } else if (s[i] == '*' || s[i] == '/') { if (!op.empty()) { while (op.top() != '+' && op.top() != '-') { char tmpop = op.top(); op.pop(); float num1 = num.top(); num.pop(); float num2 = num.top(); num.pop(); float calnum = cal(num1, num2, tmpop); num.push(calnum); } }
op.push(s[i]); } else {
float sum = 0; while (s[i] != '+' && s[i] != '-' && s[i] != '*' && s[i] != '/' && i < s.length()) { sum = sum * 10 + (s[i] - '0'); i++; } i--; num.push(sum); } } while (!op.empty()) { char tmpop = op.top(); op.pop(); float num1 = num.top(); cout<<"num1="<<num1<<endl; num.pop(); float num2 = num.top(); cout<<"num2="<<num2<<endl; num.pop(); float calnum = cal(num1, num2, tmpop); num.push(calnum); } printf("%.2f\n",num.top()); } }
|
优先队列-实现堆 (应用:哈夫曼树)
使用之前的预处理:
include<queue>
利用语句:priority_queue<int> Q;
建立一个保存元素为int的堆Q,默认为大根堆
利用语句:priority_queue<int,vector<int>,greater<int> > Q;
定义小根堆
关于堆的操作如下:
Q.push(x);
将元素x放入堆Q中
Q.top();
取出堆顶元素
Q.pop();
弹出堆顶元素,取出后堆会自动调整为一个新的小顶堆
例:
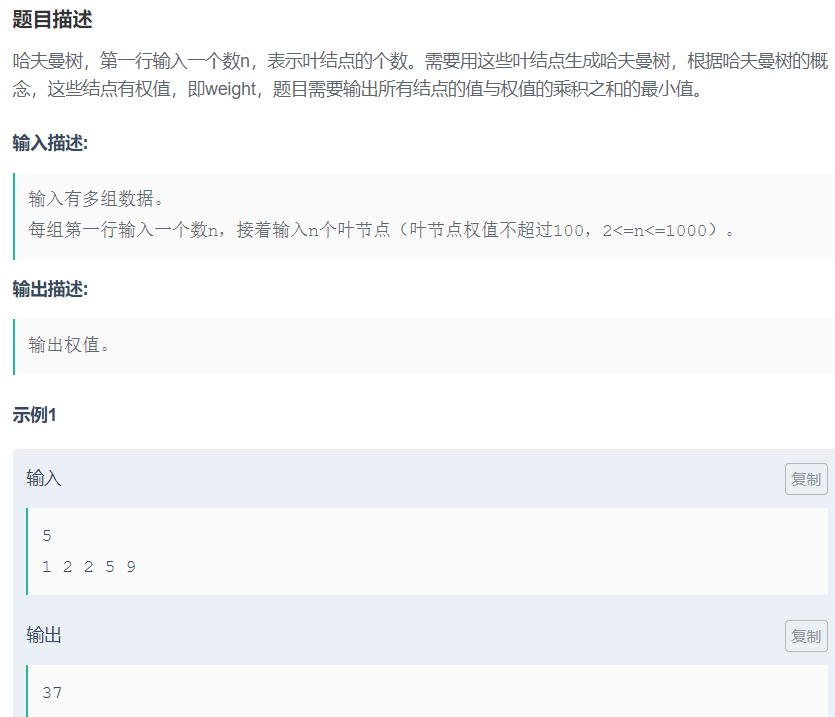
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| #include <iostream> #include <queue> using namespace std; int main() { priority_queue<int, vector<int>, greater<int> > Q; int n; while (cin >> n) { while (!Q.empty()) Q.pop(); int a; for (int i = 0; i < n; i++) { cin >> a; Q.push(a); } int ans=0; while(Q.size() >1) { int a1=Q.top(); Q.pop(); int a2=Q.top(); Q.pop(); ans+=a1+a2; Q.push(a1+a2); } cout<<ans<<endl; } return 0; }
|
map
1 2 3 4 5
| map<string,int> M;//定义一个完成从string到int映射的map M.clear();//清空一个map M.find(b);//确定map中是否保存string对象b的映射,若没有函数返回M.end() M[b]=idx;//若map中不存在string对象b的映射,则定义其映射为b映射为idx idxb=M[b];//若map中存在string对象b的映射,则读出该映射
|
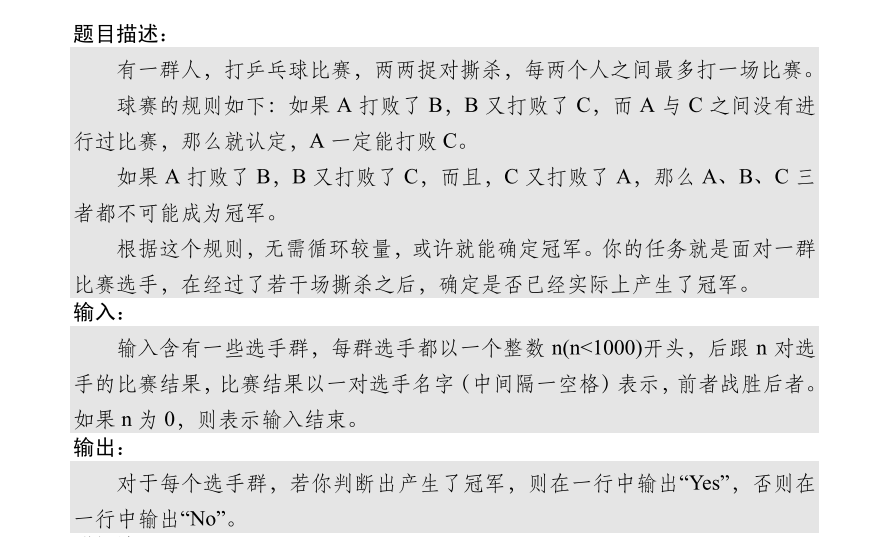
样例输入
1 2 3 4 5
| 3 Alice Bob Smith John Alice Smith 5 a c c d d e b e a d 0
|
样例输出
代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45
| #include <iostream> #include <map> #include <string> using namespace std; map<string, int> arr; int in[2002]; int main() { int n; while (cin >> n && n) { for (int i = 0; i < 2 * n; i++) in[i] = 0; string a, b; arr.clear(); int idx=0; while (n--) { cin >> a >> b; int idxa,idxb; if (arr.find(a) == arr.end()) { idxa=idx; arr[a]=idx++; } else idxa=arr[a]; if(arr.find(b)==arr.end()) { idxb=idx; arr[b]=idx++; } else idxb=arr[b]; in[idxb]++; } int cnt=0; for(int i=0;i<idx;i++) { if(in[i]==0) cnt++; } if(cnt==1) cout<<"Yes"<<endl; else cout<<"No"<<endl; } }
|